मैंने .NET में स्ट्रिंग-वैल्यू एनम बनाने के लिए बेस क्लास बनाया। यह सिर्फ एक सी # फ़ाइल है जिसे आप अपनी परियोजनाओं में कॉपी और पेस्ट कर सकते हैं, या स्ट्रिंगगैनम नामक नुगेट पैकेज के माध्यम से स्थापित कर सकते हैं ।
उपयोग:
///<completionlist cref="HexColor"/>
class HexColor : StringEnum<HexColor>
{
public static readonly HexColor Blue = New("#FF0000");
public static readonly HexColor Green = New("#00FF00");
public static readonly HexColor Red = New("#000FF");
}
विशेषताएं
- आपका StringEnum कुछ हद तक एक नियमित enum जैसा दिखता है:
// Static Parse Method
HexColor.Parse("#FF0000") // => HexColor.Red
HexColor.Parse("#ff0000", caseSensitive: false) // => HexColor.Red
HexColor.Parse("invalid") // => throws InvalidOperationException
// Static TryParse method.
HexColor.TryParse("#FF0000") // => HexColor.Red
HexColor.TryParse("#ff0000", caseSensitive: false) // => HexColor.Red
HexColor.TryParse("invalid") // => null
// Parse and TryParse returns the preexistent instances
object.ReferenceEquals(HexColor.Parse("#FF0000"), HexColor.Red) // => true
// Conversion from your `StringEnum` to `string`
string myString1 = HexColor.Red.ToString(); // => "#FF0000"
string myString2 = HexColor.Red; // => "#FF0000" (implicit cast)
- अगर xml टिप्पणी के साथ वर्ग एनोटेट किया जाता है, तो Intellisense enum नाम का सुझाव देगा
<completitionlist>
। (C # और VB दोनों में काम करता है): यानी
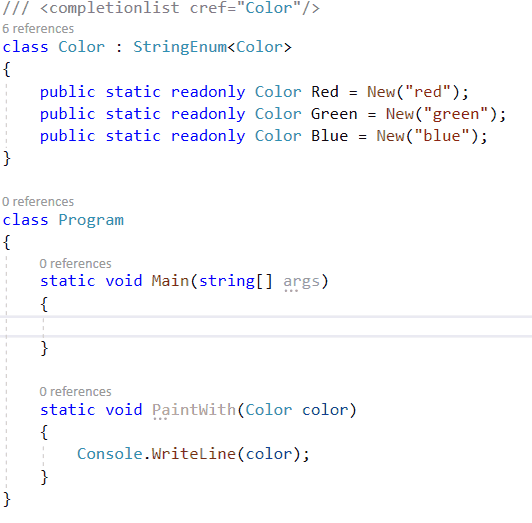
instalation
कोई एक:
- नवीनतम स्ट्रिंगनम नूगेट पैकेज स्थापित करें , जो इस पर आधारित है
.Net Standard 1.0
ताकि यह .Net Core
= = 1.0, .Net Framework
> = 4.5, Mono
> 4.6 /> आदि पर चले ।
- या अपनी परियोजना के लिए निम्न स्ट्रिंग बेस क्लास को पेस्ट करें। ( नवीनतम संस्करण )
public abstract class StringEnum<T> : IEquatable<T> where T : StringEnum<T>, new()
{
protected string Value;
private static IList<T> valueList = new List<T>();
protected static T New(string value)
{
if (value == null)
return null; // the null-valued instance is null.
var result = new T() { Value = value };
valueList.Add(result);
return result;
}
public static implicit operator string(StringEnum<T> enumValue) => enumValue.Value;
public override string ToString() => Value;
public static bool operator !=(StringEnum<T> o1, StringEnum<T> o2) => o1?.Value != o2?.Value;
public static bool operator ==(StringEnum<T> o1, StringEnum<T> o2) => o1?.Value == o2?.Value;
public override bool Equals(object other) => this.Value.Equals((other as T)?.Value ?? (other as string));
bool IEquatable<T>.Equals(T other) => this.Value.Equals(other.Value);
public override int GetHashCode() => Value.GetHashCode();
/// <summary>
/// Parse the <paramref name="value"/> specified and returns a valid <typeparamref name="T"/> or else throws InvalidOperationException.
/// </summary>
/// <param name="value">The string value representad by an instance of <typeparamref name="T"/>. Matches by string value, not by the member name.</param>
/// <param name="caseSensitive">If true, the strings must match case sensitivity.</param>
public static T Parse(string value, bool caseSensitive = false)
{
var result = TryParse(value, caseSensitive);
if (result == null)
throw new InvalidOperationException((value == null ? "null" : $"'{value}'") + $" is not a valid {typeof(T).Name}");
return result;
}
/// <summary>
/// Parse the <paramref name="value"/> specified and returns a valid <typeparamref name="T"/> or else returns null.
/// </summary>
/// <param name="value">The string value representad by an instance of <typeparamref name="T"/>. Matches by string value, not by the member name.</param>
/// <param name="caseSensitive">If true, the strings must match case sensitivity.</param>
public static T TryParse(string value, bool caseSensitive = false)
{
if (value == null) return null;
if (valueList.Count == 0) System.Runtime.CompilerServices.RuntimeHelpers.RunClassConstructor(typeof(T).TypeHandle); // force static fields initialization
var field = valueList.FirstOrDefault(f => f.Value.Equals(value,
caseSensitive ? StringComparison.Ordinal
: StringComparison.OrdinalIgnoreCase));
// Not using InvariantCulture because it's only supported in NETStandard >= 2.0
if (field == null)
return null;
return field;
}
}
- के लिए
Newtonsoft.Json
क्रमबद्धता समर्थन, बजाय इस विस्तारित संस्करण की नकल। StringEnum.cs
मुझे इस तथ्य के बाद एहसास हुआ कि यह कोड बेन के जवाब के समान है। मैंने ईमानदारी से इसे खरोंच से लिखा था। हालाँकि मुझे लगता है कि इसमें कुछ एक्स्ट्रा हैं, <completitionlist>
हैक की तरह , परिणामस्वरूप वर्ग एनम की तरह दिखता है, पार्स पर प्रतिबिंब का कोई उपयोग नहीं (), नूगेट पैकेज और रेपो जहां मैं उम्मीद करता हूं कि आने वाले मुद्दों और प्रतिक्रिया को संबोधित करेंगे।